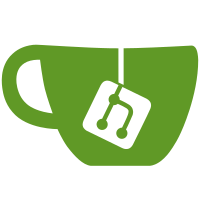
This commit doesn't actually include any direct changes to source; you have to run ./fixed-to-float.sh. Note: the script will make a number of commits itself to your git repository a various stages of the script. You will need to reset these if you want to re-run the script. * NB: Be carefull about how you reset your tree, if you are making changes to the script and patches, so you don't loose your changes * This aims to remove all use of fixed point within Clutter and Cogl. It aims to not break the Clutter API, including maintaining the CLUTTER_FIXED macros, (though they now handle floats not 16.16 fixed) It maintains cogl-fixed.[ch] as a utility API that can be used by applications (and potentially for focused internal optimisations), but all Cogl interfaces now accept floats in place of CoglFixed. Note: the choice to to use single precision floats, not doubles is very intentional. GPUs are basically all single precision; only this year have high end cards started adding double precision - aimed mostly at the GPGPU market. This means if you pass doubles into any GL[ES] driver, you can expect those numbers to be cast to a float. (Certainly this is true of Mesa wich casts most things to floats internally) It can be a noteable performance issue to cast from double->float frequently, and if we were to have an api defined in terms of doubles, that would imply a *lot* of unneeded casting. One of the noteable issues with fixed point was the amount of casting required, so I don't want to overshoot the mark and require just as much casting still. Double precision arithmatic is also slower, so it usually makes sense to minimize its use if the extra precision isn't needed. In the same way that the fast/low precision fixed API can be used sparingly for optimisations; if needs be in certain situations we can promote to doubles internally for higher precision. E.g. quoting Brian Paul (talking about performance optimisations for GL programmers): "Avoid double precision valued functions Mesa does all internal floating point computations in single precision floating point. API functions which take double precision floating point values must convert them to single precision. This can be expensive in the case of glVertex, glNormal, etc. "
41 lines
2.1 KiB
Diff
41 lines
2.1 KiB
Diff
diff --git a/clutter/clutter-actor.c b/clutter/clutter-actor.c
|
|
index ac9a2f6..42da2a5 100644
|
|
--- a/clutter/clutter-actor.c
|
|
+++ b/clutter/clutter-actor.c
|
|
@@ -866,8 +866,11 @@ clutter_actor_transform_point (ClutterActor *actor,
|
|
/* Help macros to scale from OpenGL <-1,1> coordinates system to our
|
|
* X-window based <0,window-size> coordinates
|
|
*/
|
|
-#define MTX_GL_SCALE_X(x,w,v1,v2) (CLUTTER_FIXED_MUL (((CLUTTER_FIXED_DIV ((x), (w)) + 1.0) >> 1), (v1)) + (v2))
|
|
-#define MTX_GL_SCALE_Y(y,w,v1,v2) ((v1) - CLUTTER_FIXED_MUL (((CLUTTER_FIXED_DIV ((y), (w)) + 1.0) >> 1), (v1)) + (v2))
|
|
+#define MTX_GL_SCALE_X(x,w,v1,v2) \
|
|
+ (CLUTTER_FIXED_MUL (((CLUTTER_FIXED_DIV ((x), (w)) + 1.0) / 2), (v1)) + (v2))
|
|
+#define MTX_GL_SCALE_Y(y,w,v1,v2) \
|
|
+ ((v1) - CLUTTER_FIXED_MUL (((CLUTTER_FIXED_DIV ((y), (w)) + 1.0) / 2), \
|
|
+ (v1)) + (v2))
|
|
#define MTX_GL_SCALE_Z(z,w,v1,v2) (MTX_GL_SCALE_X ((z), (w), (v1), (v2)))
|
|
|
|
/**
|
|
@@ -3213,8 +3214,8 @@ clutter_actor_get_preferred_width (ClutterActor *self,
|
|
|
|
if (natural_width < min_width)
|
|
{
|
|
- g_warning ("Actor of type %s reported a natural width of %d (%d px) "
|
|
- "lower than min width %d (%d px)",
|
|
+ g_warning ("Actor of type %s reported a natural width of %f (%d px) "
|
|
+ "lower than min width %f (%d px)",
|
|
G_OBJECT_TYPE_NAME (self),
|
|
natural_width, CLUTTER_UNITS_TO_DEVICE (natural_width),
|
|
min_width, CLUTTER_UNITS_TO_DEVICE (min_width));
|
|
@@ -3283,8 +3284,8 @@ clutter_actor_get_preferred_height (ClutterActor *self,
|
|
|
|
if (natural_height < min_height)
|
|
{
|
|
- g_warning ("Actor of type %s reported a natural height of %d "
|
|
- "(%d px) lower than min height %d (%d px)",
|
|
+ g_warning ("Actor of type %s reported a natural height of %f "
|
|
+ "(%d px) lower than min height %f (%d px)",
|
|
G_OBJECT_TYPE_NAME (self),
|
|
natural_height, CLUTTER_UNITS_TO_DEVICE (natural_height),
|
|
min_height, CLUTTER_UNITS_TO_DEVICE (min_height));
|