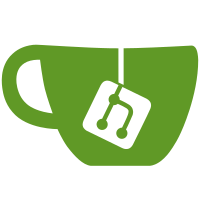
Instead of storing a pointer to the CoglRectangleMap and a handle to the atlas texture in the context, there is a now a separate data structure called a CoglAtlas to manage these two. The context just contains a pointer to this. The code to reorganise the atlas has been moved from cogl-atlas-texture.c to cogl-atlas.c
87 lines
2.7 KiB
C
87 lines
2.7 KiB
C
/*
|
|
* Cogl
|
|
*
|
|
* An object oriented GL/GLES Abstraction/Utility Layer
|
|
*
|
|
* Copyright (C) 2010 Intel Corporation.
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with this library; if not, write to the
|
|
* Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
* Boston, MA 02111-1307, USA.
|
|
*/
|
|
|
|
#ifndef __COGL_ATLAS_H
|
|
#define __COGL_ATLAS_H
|
|
|
|
#include "cogl-rectangle-map.h"
|
|
#include "cogl-callback-list.h"
|
|
|
|
typedef void
|
|
(* CoglAtlasUpdatePositionCallback) (void *user_data,
|
|
CoglHandle new_texture,
|
|
const CoglRectangleMapEntry *rect);
|
|
|
|
typedef struct _CoglAtlas CoglAtlas;
|
|
|
|
struct _CoglAtlas
|
|
{
|
|
CoglRectangleMap *map;
|
|
|
|
CoglHandle texture;
|
|
|
|
CoglAtlasUpdatePositionCallback update_position_cb;
|
|
|
|
CoglCallbackList reorganize_callbacks;
|
|
};
|
|
|
|
CoglAtlas *
|
|
_cogl_atlas_new (CoglAtlasUpdatePositionCallback update_position_cb);
|
|
|
|
gboolean
|
|
_cogl_atlas_reserve_space (CoglAtlas *atlas,
|
|
unsigned int width,
|
|
unsigned int height,
|
|
void *user_data);
|
|
|
|
void
|
|
_cogl_atlas_remove (CoglAtlas *atlas,
|
|
const CoglRectangleMapEntry *rectangle);
|
|
|
|
void
|
|
_cogl_atlas_free (CoglAtlas *atlas);
|
|
|
|
CoglHandle
|
|
_cogl_atlas_copy_rectangle (CoglAtlas *atlas,
|
|
unsigned int x,
|
|
unsigned int y,
|
|
unsigned int width,
|
|
unsigned int height,
|
|
CoglTextureFlags flags,
|
|
CoglPixelFormat format);
|
|
|
|
CoglAtlas *
|
|
_cogl_atlas_get_default (void);
|
|
|
|
void
|
|
_cogl_atlas_add_reorganize_callback (CoglAtlas *atlas,
|
|
CoglCallbackListFunc callback,
|
|
void *user_data);
|
|
|
|
void
|
|
_cogl_atlas_remove_reorganize_callback (CoglAtlas *atlas,
|
|
CoglCallbackListFunc callback,
|
|
void *user_data);
|
|
|
|
#endif /* __COGL_ATLAS_H */
|