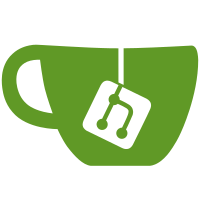
This uses the queue that was introduced when migrating impl task management from MetaThread to MetaThreadImpl, with the exception that it's now fully used as an actual queue. It now has a GSource that sits on the right GMainContext that is dispatched whenever there are tasks to execute. Part-of: <https://gitlab.gnome.org/GNOME/mutter/-/merge_requests/2777>
89 lines
3.3 KiB
C
89 lines
3.3 KiB
C
/*
|
|
* Copyright (C) 2018-2021 Red Hat
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License as
|
|
* published by the Free Software Foundation; either version 2 of the
|
|
* License, or (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful, but
|
|
* WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA
|
|
* 02111-1307, USA.
|
|
*/
|
|
|
|
#ifndef META_THREAD_H
|
|
#define META_THREAD_H
|
|
|
|
#include <glib-object.h>
|
|
|
|
#include "backends/meta-backend-types.h"
|
|
#include "core/util-private.h"
|
|
|
|
typedef struct _MetaThreadImpl MetaThreadImpl;
|
|
|
|
#define META_TYPE_THREAD (meta_thread_get_type ())
|
|
META_EXPORT_TEST
|
|
G_DECLARE_DERIVABLE_TYPE (MetaThread, meta_thread,
|
|
META, THREAD,
|
|
GObject)
|
|
|
|
struct _MetaThreadClass
|
|
{
|
|
GObjectClass parent_class;
|
|
};
|
|
|
|
typedef void (* MetaThreadCallback) (MetaThread *thread,
|
|
gpointer user_data);
|
|
|
|
typedef gpointer (* MetaThreadTaskFunc) (MetaThreadImpl *thread_impl,
|
|
gpointer user_data,
|
|
GError **error);
|
|
typedef void (* MetaThreadTaskFeedbackFunc) (gpointer retval,
|
|
const GError *error,
|
|
gpointer user_data);
|
|
|
|
META_EXPORT_TEST
|
|
void meta_thread_queue_callback (MetaThread *thread,
|
|
MetaThreadCallback callback,
|
|
gpointer user_data,
|
|
GDestroyNotify user_data_destroy);
|
|
|
|
META_EXPORT_TEST
|
|
int meta_thread_flush_callbacks (MetaThread *thread);
|
|
|
|
META_EXPORT_TEST
|
|
gpointer meta_thread_run_impl_task_sync (MetaThread *thread,
|
|
MetaThreadTaskFunc func,
|
|
gpointer user_data,
|
|
GError **error);
|
|
|
|
META_EXPORT_TEST
|
|
void meta_thread_post_impl_task (MetaThread *thread,
|
|
MetaThreadTaskFunc func,
|
|
gpointer user_data,
|
|
MetaThreadTaskFeedbackFunc feedback_func,
|
|
gpointer feedback_user_data);
|
|
|
|
META_EXPORT_TEST
|
|
MetaBackend * meta_thread_get_backend (MetaThread *thread);
|
|
|
|
META_EXPORT_TEST
|
|
gboolean meta_thread_is_in_impl_task (MetaThread *thread);
|
|
|
|
gboolean meta_thread_is_waiting_for_impl_task (MetaThread *thread);
|
|
|
|
#define meta_assert_in_thread_impl(thread) \
|
|
g_assert (meta_thread_is_in_impl_task (thread))
|
|
#define meta_assert_not_in_thread_impl(thread) \
|
|
g_assert (!meta_thread_is_in_impl_task (thread))
|
|
#define meta_assert_is_waiting_for_thread_impl_task(thread) \
|
|
g_assert (meta_thread_is_waiting_for_impl_task (thread))
|
|
|
|
#endif /* META_THREAD_H */
|