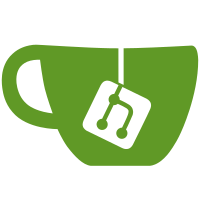
When computing the pixels value of a ClutterUnits value we should be caching the value to avoid recomputing for every call of clutter_units_to_pixels(). We already have a flag telling us to return the cached value, but we miss the mechanism to evict the cache whenever the Backend settings affecting the conversion, that is default font and resolution, change. In order to implement the eviction we can use a "serial"; the Backend will have an internal serial field which we retrieve and put inside the ClutterUnits structure (we split one of the two 64 bit padding fields into two 32 bit fields to maintain ABI); every time we call clutter_units_to_pixels() we compare the units serial with that of the Backend; if they match and pixels_set is set to TRUE then we just return the stored pixels value. If the serials do not match then we unset the pixels_set flag and recompute the pixels value. We can verify this by adding a simple test unit checking that by changing the resolution of ClutterBackend we get different pixel values for 1 em. http://bugzilla.openedhand.com/show_bug.cgi?id=1843
119 lines
4.3 KiB
C
119 lines
4.3 KiB
C
#include <stdio.h>
|
|
#include <clutter/clutter.h>
|
|
|
|
#include "test-conform-common.h"
|
|
|
|
void
|
|
test_units_cache (TestConformSimpleFixture *fixture,
|
|
gconstpointer data)
|
|
{
|
|
ClutterUnits units;
|
|
ClutterBackend *backend;
|
|
gfloat pixels;
|
|
gdouble dpi;
|
|
|
|
backend = clutter_get_default_backend ();
|
|
|
|
dpi = clutter_backend_get_resolution (backend);
|
|
|
|
clutter_units_from_em (&units, 1.0);
|
|
pixels = clutter_units_to_pixels (&units);
|
|
|
|
clutter_backend_set_resolution (backend, dpi + 10);
|
|
g_assert_cmpfloat (clutter_units_to_pixels (&units), !=, pixels);
|
|
|
|
clutter_backend_set_resolution (backend, dpi);
|
|
g_assert_cmpfloat (clutter_units_to_pixels (&units), ==, pixels);
|
|
}
|
|
|
|
void
|
|
test_units_constructors (TestConformSimpleFixture *fixture,
|
|
gconstpointer data)
|
|
{
|
|
ClutterUnits units, units_cm;
|
|
|
|
clutter_units_from_pixels (&units, 100);
|
|
g_assert (clutter_units_get_unit_type (&units) == CLUTTER_UNIT_PIXEL);
|
|
g_assert_cmpfloat (clutter_units_get_unit_value (&units), ==, 100.0);
|
|
g_assert_cmpfloat (clutter_units_to_pixels (&units), ==, 100.0);
|
|
|
|
clutter_units_from_em (&units, 5.0);
|
|
g_assert (clutter_units_get_unit_type (&units) == CLUTTER_UNIT_EM);
|
|
g_assert_cmpfloat (clutter_units_get_unit_value (&units), ==, 5.0);
|
|
g_assert_cmpfloat (clutter_units_to_pixels (&units), !=, 5.0);
|
|
|
|
clutter_units_from_cm (&units_cm, 5.0);
|
|
g_assert (clutter_units_get_unit_type (&units_cm) == CLUTTER_UNIT_CM);
|
|
g_assert_cmpfloat (clutter_units_get_unit_value (&units_cm), ==, 5.0);
|
|
g_assert_cmpfloat (clutter_units_to_pixels (&units_cm), !=, 5.0);
|
|
|
|
clutter_units_from_mm (&units, 50.0);
|
|
g_assert (clutter_units_get_unit_type (&units) == CLUTTER_UNIT_MM);
|
|
g_assert_cmpfloat (clutter_units_to_pixels (&units),
|
|
==,
|
|
clutter_units_to_pixels (&units_cm));
|
|
}
|
|
|
|
void
|
|
test_units_string (TestConformSimpleFixture *fixture,
|
|
gconstpointer data)
|
|
{
|
|
ClutterUnits units;
|
|
gchar *string;
|
|
|
|
g_assert (clutter_units_from_string (&units, "10") == TRUE);
|
|
g_assert (clutter_units_get_unit_type (&units) == CLUTTER_UNIT_PIXEL);
|
|
g_assert_cmpfloat (clutter_units_get_unit_value (&units), ==, 10);
|
|
|
|
g_assert (clutter_units_from_string (&units, "10 ") == TRUE);
|
|
g_assert (clutter_units_get_unit_type (&units) == CLUTTER_UNIT_PIXEL);
|
|
g_assert_cmpfloat (clutter_units_get_unit_value (&units), ==, 10);
|
|
|
|
g_assert (clutter_units_from_string (&units, "5 em") == TRUE);
|
|
g_assert (clutter_units_get_unit_type (&units) == CLUTTER_UNIT_EM);
|
|
g_assert_cmpfloat (clutter_units_get_unit_value (&units), ==, 5);
|
|
|
|
g_assert (clutter_units_from_string (&units, "5 emeralds") == FALSE);
|
|
|
|
g_assert (clutter_units_from_string (&units, " 16 mm") == TRUE);
|
|
g_assert (clutter_units_get_unit_type (&units) == CLUTTER_UNIT_MM);
|
|
g_assert_cmpfloat (clutter_units_get_unit_value (&units), ==, 16);
|
|
|
|
g_assert (clutter_units_from_string (&units, " 24 pt ") == TRUE);
|
|
g_assert (clutter_units_get_unit_type (&units) == CLUTTER_UNIT_POINT);
|
|
g_assert_cmpfloat (clutter_units_get_unit_value (&units), ==, 24);
|
|
|
|
g_assert (clutter_units_from_string (&units, " 32 em garbage") == FALSE);
|
|
|
|
g_assert (clutter_units_from_string (&units, "5.1cm") == TRUE);
|
|
g_assert (clutter_units_get_unit_type (&units) == CLUTTER_UNIT_CM);
|
|
g_assert_cmpfloat (clutter_units_get_unit_value (&units), ==, 5.1f);
|
|
|
|
g_assert (clutter_units_from_string (&units, "5,mm") == FALSE);
|
|
|
|
g_assert (clutter_units_from_string (&units, ".5pt") == TRUE);
|
|
g_assert (clutter_units_get_unit_type (&units) == CLUTTER_UNIT_POINT);
|
|
g_assert_cmpfloat (clutter_units_get_unit_value (&units), ==, 0.5f);
|
|
|
|
g_assert (clutter_units_from_string (&units, "1 omg!!pony") == FALSE);
|
|
|
|
clutter_units_from_pt (&units, 24.0);
|
|
string = clutter_units_to_string (&units);
|
|
g_assert_cmpstr (string, ==, "24.0 pt");
|
|
g_free (string);
|
|
|
|
clutter_units_from_em (&units, 3.0);
|
|
string = clutter_units_to_string (&units);
|
|
g_assert_cmpstr (string, ==, "3.00 em");
|
|
|
|
units.unit_type = CLUTTER_UNIT_PIXEL;
|
|
units.value = 0;
|
|
|
|
g_assert (clutter_units_from_string (&units, string) == TRUE);
|
|
g_assert (clutter_units_get_unit_type (&units) != CLUTTER_UNIT_PIXEL);
|
|
g_assert (clutter_units_get_unit_type (&units) == CLUTTER_UNIT_EM);
|
|
g_assert_cmpint ((int) clutter_units_get_unit_value (&units), ==, 3);
|
|
|
|
g_free (string);
|
|
}
|