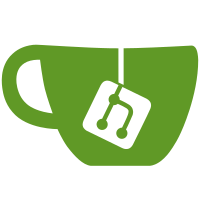
In order to make things more and more asynchronus and to each time we paint be an isolated event, that can be potentially be applied individually or together with other updates, make it so that each time we draw, we use the transient MetaFrameNative (ClutterFrame) instance to carry a KMS update for us. For this to work, we also need to restructure how we apply mode sets. Previously we'd amend the same KMS update each frame during mode set, then after the last CRTC was composited, we'd apply the update that contained updates for all CRTC. Now each CRTC has its own KMS update, and instead we put them in a per device table, and whenever we finished painting, we'll merge the new update into any existing one, and then finally once all CRTCs have been composited, we'll apply an update that contains all the mode sets for all relevant CRTCs on a device. Part-of: <https://gitlab.gnome.org/GNOME/mutter/-/merge_requests/2855>
109 lines
4.6 KiB
C
109 lines
4.6 KiB
C
/*
|
|
* Copyright (C) 2011 Intel Corporation.
|
|
* Copyright (C) 2016-2020 Red Hat
|
|
* Copyright (c) 2018,2019 DisplayLink (UK) Ltd.
|
|
*
|
|
* Permission is hereby granted, free of charge, to any person
|
|
* obtaining a copy of this software and associated documentation
|
|
* files (the "Software"), to deal in the Software without
|
|
* restriction, including without limitation the rights to use, copy,
|
|
* modify, merge, publish, distribute, sublicense, and/or sell copies
|
|
* of the Software, and to permit persons to whom the Software is
|
|
* furnished to do so, subject to the following conditions:
|
|
*
|
|
* The above copyright notice and this permission notice shall be
|
|
* included in all copies or substantial portions of the Software.
|
|
*
|
|
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
|
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
|
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
|
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS
|
|
* BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN
|
|
* ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN
|
|
* CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
|
* SOFTWARE.
|
|
*
|
|
*/
|
|
|
|
#ifndef META_RENDERER_NATIVE_PRIVATE_H
|
|
#define META_RENDERER_NATIVE_PRIVATE_H
|
|
|
|
#include "backends/meta-gles3.h"
|
|
#include "backends/native/meta-backend-native-types.h"
|
|
#include "backends/native/meta-renderer-native.h"
|
|
|
|
typedef enum _MetaSharedFramebufferCopyMode
|
|
{
|
|
/* Zero-copy: primary GPU exports, secondary GPU imports as KMS FB */
|
|
META_SHARED_FRAMEBUFFER_COPY_MODE_ZERO,
|
|
/* the secondary GPU will make the copy */
|
|
META_SHARED_FRAMEBUFFER_COPY_MODE_SECONDARY_GPU,
|
|
/*
|
|
* The copy is made in the primary GPU rendering context, either
|
|
* as a CPU copy through Cogl read-pixels or as primary GPU copy
|
|
* using glBlitFramebuffer.
|
|
*/
|
|
META_SHARED_FRAMEBUFFER_COPY_MODE_PRIMARY
|
|
} MetaSharedFramebufferCopyMode;
|
|
|
|
typedef struct _MetaRendererNativeGpuData
|
|
{
|
|
MetaRendererNative *renderer_native;
|
|
|
|
MetaRenderDevice *render_device;
|
|
MetaGpuKms *gpu_kms;
|
|
|
|
MetaRendererNativeMode mode;
|
|
|
|
/*
|
|
* Fields used for blitting iGPU framebuffer content onto dGPU framebuffers.
|
|
*/
|
|
struct {
|
|
MetaSharedFramebufferCopyMode copy_mode;
|
|
gboolean has_EGL_EXT_image_dma_buf_import_modifiers;
|
|
|
|
/* For GPU blit mode */
|
|
EGLContext egl_context;
|
|
EGLConfig egl_config;
|
|
} secondary;
|
|
} MetaRendererNativeGpuData;
|
|
|
|
MetaEgl * meta_renderer_native_get_egl (MetaRendererNative *renderer_native);
|
|
|
|
MetaGles3 * meta_renderer_native_get_gles3 (MetaRendererNative *renderer_native);
|
|
|
|
MetaRendererNativeGpuData * meta_renderer_native_get_gpu_data (MetaRendererNative *renderer_native,
|
|
MetaGpuKms *gpu_kms);
|
|
|
|
META_EXPORT_TEST
|
|
gboolean meta_renderer_native_has_pending_mode_sets (MetaRendererNative *renderer_native);
|
|
|
|
gboolean meta_renderer_native_has_pending_mode_set (MetaRendererNative *renderer_native);
|
|
|
|
void meta_renderer_native_notify_mode_sets_reset (MetaRendererNative *renderer_native);
|
|
|
|
void meta_renderer_native_post_mode_set_updates (MetaRendererNative *renderer_native);
|
|
|
|
void meta_renderer_native_queue_mode_set_update (MetaRendererNative *renderer_native,
|
|
MetaKmsUpdate *new_kms_update);
|
|
|
|
void meta_renderer_native_queue_power_save_page_flip (MetaRendererNative *renderer_native,
|
|
CoglOnscreen *onscreen);
|
|
|
|
CoglFramebuffer * meta_renderer_native_create_dma_buf_framebuffer (MetaRendererNative *renderer_native,
|
|
int dmabuf_fd,
|
|
uint32_t width,
|
|
uint32_t height,
|
|
uint32_t stride,
|
|
uint32_t offset,
|
|
uint64_t modifier,
|
|
uint32_t drm_format,
|
|
GError **error);
|
|
|
|
gboolean meta_renderer_native_pop_pending_mode_set (MetaRendererNative *renderer_native,
|
|
MetaRendererView *view);
|
|
|
|
const CoglWinsysVtable * meta_get_renderer_native_parent_vtable (void);
|
|
|
|
#endif /* META_RENDERER_NATIVE_PRIVATE_H */
|