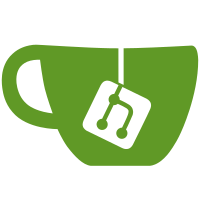
Threaded swap wait was added for using together with the Nvidia GLX driver due to the lack of anything equivalent to the INTEL_swap_event GLX extension. The purpose was to avoid inhibiting the invocation of idle callbacks when constantly rendering, as the combination of throttling on swap-interval 1 and glxSwapBuffers() and the frame clock source having higher priority than the default idle callback sources meant they would never be invoked. This was solved in gbz#779039 by introducing a thread that took care of the vsync waiting, pushing frame completion events to the main thread meaning the main thread could go idle while waiting to draw the next frame instead of blocking on glxSwapBuffers(). As of https://gitlab.gnome.org/GNOME/mutter/merge_requests/363, the main thread will instead use prediction to estimate when the next frame should be drawn. A side effect of this is that even without INTEL_swap_event, we would not block as much, or at all, on glxSwapBuffers(), as at the time it is called, we have likely already hit the vblank, or will hit it soon. After having introduced the swap waiting thread, it was observed that the Nvidia driver used a considerable amount of CPU waiting for the vblank, effectively wasting CPU time. The need to call glFinish() was also problematic as it would wait for the frame to finish, before continuing. Due to this, remove the threaded swap wait, and rely only on the frame clock not scheduling frames too early. Fixes: https://bugzilla.gnome.org/show_bug.cgi?id=781835 Related: https://gitlab.gnome.org/GNOME/mutter/issues/700 [jadahl: Rewrote commit message] https://gitlab.gnome.org/GNOME/mutter/merge_requests/602
119 lines
3.8 KiB
C
119 lines
3.8 KiB
C
/*
|
|
* Cogl
|
|
*
|
|
* A Low Level GPU Graphics and Utilities API
|
|
*
|
|
* Copyright (C) 2010,2013 Intel Corporation.
|
|
*
|
|
* Permission is hereby granted, free of charge, to any person
|
|
* obtaining a copy of this software and associated documentation
|
|
* files (the "Software"), to deal in the Software without
|
|
* restriction, including without limitation the rights to use, copy,
|
|
* modify, merge, publish, distribute, sublicense, and/or sell copies
|
|
* of the Software, and to permit persons to whom the Software is
|
|
* furnished to do so, subject to the following conditions:
|
|
*
|
|
* The above copyright notice and this permission notice shall be
|
|
* included in all copies or substantial portions of the Software.
|
|
*
|
|
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
|
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
|
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
|
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS
|
|
* BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN
|
|
* ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN
|
|
* CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
|
* SOFTWARE.
|
|
*
|
|
*
|
|
*/
|
|
|
|
#ifndef __COGL_PRIVATE_H__
|
|
#define __COGL_PRIVATE_H__
|
|
|
|
#include <cogl/cogl-pipeline.h>
|
|
|
|
#include "cogl-context.h"
|
|
#include "cogl-flags.h"
|
|
|
|
G_BEGIN_DECLS
|
|
|
|
typedef enum
|
|
{
|
|
COGL_PRIVATE_FEATURE_TEXTURE_2D_FROM_EGL_IMAGE,
|
|
COGL_PRIVATE_FEATURE_MESA_PACK_INVERT,
|
|
COGL_PRIVATE_FEATURE_BLIT_FRAMEBUFFER,
|
|
COGL_PRIVATE_FEATURE_FOUR_CLIP_PLANES,
|
|
COGL_PRIVATE_FEATURE_PBOS,
|
|
COGL_PRIVATE_FEATURE_VBOS,
|
|
COGL_PRIVATE_FEATURE_EXT_PACKED_DEPTH_STENCIL,
|
|
COGL_PRIVATE_FEATURE_OES_PACKED_DEPTH_STENCIL,
|
|
COGL_PRIVATE_FEATURE_TEXTURE_FORMAT_BGRA8888,
|
|
COGL_PRIVATE_FEATURE_UNPACK_SUBIMAGE,
|
|
COGL_PRIVATE_FEATURE_SAMPLER_OBJECTS,
|
|
COGL_PRIVATE_FEATURE_READ_PIXELS_ANY_FORMAT,
|
|
COGL_PRIVATE_FEATURE_ALPHA_TEST,
|
|
COGL_PRIVATE_FEATURE_FORMAT_CONVERSION,
|
|
COGL_PRIVATE_FEATURE_QUADS,
|
|
COGL_PRIVATE_FEATURE_BLEND_CONSTANT,
|
|
COGL_PRIVATE_FEATURE_QUERY_FRAMEBUFFER_BITS,
|
|
COGL_PRIVATE_FEATURE_BUILTIN_POINT_SIZE_UNIFORM,
|
|
COGL_PRIVATE_FEATURE_QUERY_TEXTURE_PARAMETERS,
|
|
COGL_PRIVATE_FEATURE_ALPHA_TEXTURES,
|
|
COGL_PRIVATE_FEATURE_TEXTURE_SWIZZLE,
|
|
COGL_PRIVATE_FEATURE_TEXTURE_MAX_LEVEL,
|
|
COGL_PRIVATE_FEATURE_OES_EGL_SYNC,
|
|
/* If this is set then the winsys is responsible for queueing dirty
|
|
* events. Otherwise a dirty event will be queued when the onscreen
|
|
* is first allocated or when it is shown or resized */
|
|
COGL_PRIVATE_FEATURE_DIRTY_EVENTS,
|
|
COGL_PRIVATE_FEATURE_ENABLE_PROGRAM_POINT_SIZE,
|
|
/* These features let us avoid conditioning code based on the exact
|
|
* driver being used and instead check for broad opengl feature
|
|
* sets that can be shared by several GL apis */
|
|
COGL_PRIVATE_FEATURE_ANY_GL,
|
|
COGL_PRIVATE_FEATURE_GL_FIXED,
|
|
COGL_PRIVATE_FEATURE_GL_PROGRAMMABLE,
|
|
COGL_PRIVATE_FEATURE_GL_EMBEDDED,
|
|
COGL_PRIVATE_FEATURE_GL_WEB,
|
|
|
|
COGL_N_PRIVATE_FEATURES
|
|
} CoglPrivateFeature;
|
|
|
|
/* Sometimes when evaluating pipelines, either during comparisons or
|
|
* if calculating a hash value we need to tweak the evaluation
|
|
* semantics */
|
|
typedef enum _CoglPipelineEvalFlags
|
|
{
|
|
COGL_PIPELINE_EVAL_FLAG_NONE = 0
|
|
} CoglPipelineEvalFlags;
|
|
|
|
void
|
|
_cogl_transform_point (const CoglMatrix *matrix_mv,
|
|
const CoglMatrix *matrix_p,
|
|
const float *viewport,
|
|
float *x,
|
|
float *y);
|
|
|
|
gboolean
|
|
_cogl_check_extension (const char *name, char * const *ext);
|
|
|
|
void
|
|
_cogl_clear (const CoglColor *color, unsigned long buffers);
|
|
|
|
void
|
|
_cogl_init (void);
|
|
|
|
void
|
|
_cogl_push_source (CoglPipeline *pipeline, gboolean enable_legacy);
|
|
|
|
gboolean
|
|
_cogl_get_enable_legacy_state (void);
|
|
|
|
#define _cogl_has_private_feature(ctx, feature) \
|
|
COGL_FLAGS_GET ((ctx)->private_features, (feature))
|
|
|
|
G_END_DECLS
|
|
|
|
#endif /* __COGL_PRIVATE_H__ */
|