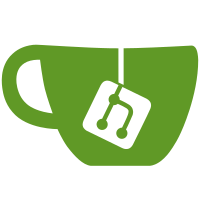
The features_cached member of CoglContext is intended to mark when we've calculated the features so that we know if they are ready in cogl_get_features. However we always intialize the features while creating the context so features_cached will never be FALSE so it's not useful. We also had the odd behaviour that the COGL_DEBUG feature overrides were only applied in the first call to cogl_get_features. However there are other functions that use the feature flags such as cogl_features_available that don't use this function so in some cases the feature flags will be interpreted before the overrides are applied. This patch makes it always initialize the features and apply the overrides immediately while creating the context. This fixes a problem with COGL_DEBUG=disable-arbfp where the first material flushed is done before any call to cogl_get_features so it may still use ARBfp.
122 lines
4.0 KiB
C
122 lines
4.0 KiB
C
/*
|
|
* Cogl
|
|
*
|
|
* An object oriented GL/GLES Abstraction/Utility Layer
|
|
*
|
|
* Copyright (C) 2007,2008,2009 Intel Corporation.
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with this library. If not, see <http://www.gnu.org/licenses/>.
|
|
*
|
|
*
|
|
*/
|
|
|
|
#ifdef HAVE_CONFIG_H
|
|
#include "config.h"
|
|
#endif
|
|
|
|
#include <string.h>
|
|
|
|
#include "cogl.h"
|
|
#include "cogl-internal.h"
|
|
#include "cogl-context.h"
|
|
#include "cogl-feature-private.h"
|
|
|
|
gboolean
|
|
_cogl_check_driver_valid (GError **error)
|
|
{
|
|
/* The GLES backend doesn't have any particular version requirements */
|
|
return TRUE;
|
|
}
|
|
|
|
/* Define a set of arrays containing the functions required from GL
|
|
for each feature */
|
|
#define COGL_FEATURE_BEGIN(name, min_gl_major, min_gl_minor, \
|
|
namespaces, extension_names, \
|
|
feature_flags, feature_flags_private) \
|
|
static const CoglFeatureFunction cogl_feature_ ## name ## _funcs[] = {
|
|
#define COGL_FEATURE_FUNCTION(ret, name, args) \
|
|
{ G_STRINGIFY (name), G_STRUCT_OFFSET (CoglContext, drv.pf_ ## name) },
|
|
#define COGL_FEATURE_END() \
|
|
{ NULL, 0 }, \
|
|
};
|
|
#include "cogl-feature-functions-gles.h"
|
|
|
|
/* Define an array of features */
|
|
#undef COGL_FEATURE_BEGIN
|
|
#define COGL_FEATURE_BEGIN(name, min_gl_major, min_gl_minor, \
|
|
namespaces, extension_names, \
|
|
feature_flags, feature_flags_private) \
|
|
{ min_gl_major, min_gl_minor, namespaces, \
|
|
extension_names, feature_flags, feature_flags_private, \
|
|
cogl_feature_ ## name ## _funcs },
|
|
#undef COGL_FEATURE_FUNCTION
|
|
#define COGL_FEATURE_FUNCTION(ret, name, args)
|
|
#undef COGL_FEATURE_END
|
|
#define COGL_FEATURE_END()
|
|
|
|
static const CoglFeatureData cogl_feature_data[] =
|
|
{
|
|
#include "cogl-feature-functions-gles.h"
|
|
};
|
|
|
|
void
|
|
_cogl_features_init (void)
|
|
{
|
|
CoglFeatureFlags flags = 0;
|
|
int max_clip_planes = 0;
|
|
GLint num_stencil_bits = 0;
|
|
const char *gl_extensions;
|
|
int i;
|
|
|
|
_COGL_GET_CONTEXT (ctx, NO_RETVAL);
|
|
|
|
gl_extensions = (const char*) glGetString (GL_EXTENSIONS);
|
|
|
|
for (i = 0; i < G_N_ELEMENTS (cogl_feature_data); i++)
|
|
if (_cogl_feature_check ("GL", cogl_feature_data + i,
|
|
0, 0,
|
|
gl_extensions))
|
|
flags |= cogl_feature_data[i].feature_flags;
|
|
|
|
GE( glGetIntegerv (GL_STENCIL_BITS, &num_stencil_bits) );
|
|
/* We need at least three stencil bits to combine clips */
|
|
if (num_stencil_bits > 2)
|
|
flags |= COGL_FEATURE_STENCIL_BUFFER;
|
|
|
|
GE( glGetIntegerv (GL_MAX_CLIP_PLANES, &max_clip_planes) );
|
|
if (max_clip_planes >= 4)
|
|
flags |= COGL_FEATURE_FOUR_CLIP_PLANES;
|
|
|
|
#ifdef HAVE_COGL_GLES2
|
|
flags |= COGL_FEATURE_SHADERS_GLSL | COGL_FEATURE_OFFSCREEN;
|
|
/* Note GLES 2 core doesn't support mipmaps for npot textures or
|
|
* repeat modes other than CLAMP_TO_EDGE. */
|
|
flags |= COGL_FEATURE_TEXTURE_NPOT_BASIC;
|
|
#endif
|
|
|
|
/* FIXME: HACK: We are in the process of overhauling the GLES 2 backend
|
|
* and consolidating with a CoglMaterial GLSL backend. Currently though
|
|
* use of CoglBuffers with GLES 2 is broken. */
|
|
#ifndef HAVE_COGL_GLES2
|
|
flags |= COGL_FEATURE_VBOS;
|
|
#endif
|
|
|
|
/* Both GLES 1.1 and GLES 2.0 support point sprites in core */
|
|
flags |= COGL_FEATURE_POINT_SPRITE;
|
|
|
|
/* Cache features */
|
|
ctx->feature_flags = flags;
|
|
}
|
|
|