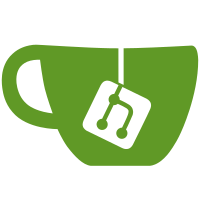
We now prepend a set of defines to any given GLSL shader so that we can define builtin uniforms/attributes within the "cogl" namespace that we can use to provide compatibility across a range of the earlier versions of GLSL. This updates test-cogl-shader-glsl.c and test-shader.c so they no longer needs to special case GLES vs GL when splicing together its shaders as well as the blur, colorize and desaturate effects. To get a feel for the new, portable uniform/attribute names here are the defines for OpenGL vertex shaders: #define cogl_position_in gl_Vertex #define cogl_color_in gl_Color #define cogl_tex_coord_in gl_MultiTexCoord0 #define cogl_tex_coord0_in gl_MultiTexCoord0 #define cogl_tex_coord1_in gl_MultiTexCoord1 #define cogl_tex_coord2_in gl_MultiTexCoord2 #define cogl_tex_coord3_in gl_MultiTexCoord3 #define cogl_tex_coord4_in gl_MultiTexCoord4 #define cogl_tex_coord5_in gl_MultiTexCoord5 #define cogl_tex_coord6_in gl_MultiTexCoord6 #define cogl_tex_coord7_in gl_MultiTexCoord7 #define cogl_normal_in gl_Normal #define cogl_position_out gl_Position #define cogl_point_size_out gl_PointSize #define cogl_color_out gl_FrontColor #define cogl_tex_coord_out gl_TexCoord #define cogl_modelview_matrix gl_ModelViewMatrix #define cogl_modelview_projection_matrix gl_ModelViewProjectionMatrix #define cogl_projection_matrix gl_ProjectionMatrix #define cogl_texture_matrix gl_TextureMatrix And for fragment shaders we have: #define cogl_color_in gl_Color #define cogl_tex_coord_in gl_TexCoord #define cogl_color_out gl_FragColor #define cogl_depth_out gl_FragDepth #define cogl_front_facing gl_FrontFacing
74 lines
2.1 KiB
GLSL
74 lines
2.1 KiB
GLSL
/*** _cogl_fixed_vertex_shader_per_vertex_attribs ***/
|
|
|
|
/* Per vertex attributes */
|
|
attribute vec4 cogl_position_in;
|
|
attribute vec4 cogl_color_in;
|
|
|
|
/*** _cogl_fixed_vertex_shader_transform_matrices ***/
|
|
|
|
/* Transformation matrices */
|
|
uniform mat4 cogl_modelview_matrix;
|
|
uniform mat4 cogl_modelview_projection_matrix; /* combined modelview and projection matrix */
|
|
|
|
/*** _cogl_fixed_vertex_shader_output_variables ***/
|
|
|
|
/* Outputs to the fragment shader */
|
|
varying vec4 _cogl_color;
|
|
varying float _cogl_fog_amount;
|
|
|
|
/*** _cogl_fixed_vertex_shader_fogging_options ***/
|
|
|
|
/* Fogging options */
|
|
uniform float _cogl_fog_density;
|
|
uniform float _cogl_fog_start;
|
|
uniform float _cogl_fog_end;
|
|
|
|
/* Point options */
|
|
uniform float cogl_point_size_in;
|
|
|
|
/*** _cogl_fixed_vertex_shader_main_start ***/
|
|
|
|
void
|
|
main (void)
|
|
{
|
|
vec4 transformed_tex_coord;
|
|
|
|
/* Calculate the transformed position */
|
|
gl_Position = cogl_modelview_projection_matrix * cogl_position_in;
|
|
|
|
/* Copy across the point size from the uniform */
|
|
gl_PointSize = cogl_point_size_in;
|
|
|
|
/* Calculate the transformed texture coordinate */
|
|
|
|
/*** _cogl_fixed_vertex_shader_frag_color_start ***/
|
|
|
|
/* Pass the interpolated vertex color on to the fragment shader */
|
|
_cogl_color = cogl_color_in;
|
|
|
|
/*** _cogl_fixed_vertex_shader_fog_start ***/
|
|
|
|
/* Estimate the distance from the eye using just the z-coordinate to
|
|
use as the fog coord */
|
|
vec4 eye_coord = cogl_modelview_matrix * cogl_position_in;
|
|
float fog_coord = abs (eye_coord.z / eye_coord.w);
|
|
|
|
/* Calculate the fog amount per-vertex and interpolate it for the
|
|
fragment shader */
|
|
|
|
/*** _cogl_fixed_vertex_shader_fog_exp ***/
|
|
_cogl_fog_amount = exp (-fog_density * fog_coord);
|
|
/*** _cogl_fixed_vertex_shader_fog_exp2 ***/
|
|
_cogl_fog_amount = exp (-_cogl_fog_density * fog_coord
|
|
* _cogl_fog_density * fog_coord);
|
|
/*** _cogl_fixed_vertex_shader_fog_linear ***/
|
|
_cogl_fog_amount = (_cogl_fog_end - fog_coord) /
|
|
(_cogl_fog_end - _cogl_fog_start);
|
|
|
|
/*** _cogl_fixed_vertex_shader_fog_end ***/
|
|
_cogl_fog_amount = clamp (_cogl_fog_amount, 0.0, 1.0);
|
|
|
|
/*** _cogl_fixed_vertex_shader_end ***/
|
|
}
|
|
|