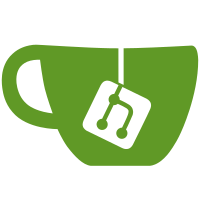
This adds three new internal API functions which can be used to retain the clip stack state and restore it later: _cogl_get_clip_stack _cogl_set_clip_stack _cogl_clip_stack_copy The functions are currently internal and not yet used but we may want to make them public in future to replace the cogl_clip_stack_save() and cogl_clip_stack_restore() APIs. The get function just returns the handle to the clip stack at the top of the stack of stacks and the set function just replaces it. The copy function makes a cheap copy of an existing stack by taking a reference to the top stack entry. This ends up working like a deep copy because there is no way to modify entries of a stack but it doesn't actually copy the data.
76 lines
2.4 KiB
C
76 lines
2.4 KiB
C
/*
|
|
* Cogl
|
|
*
|
|
* An object oriented GL/GLES Abstraction/Utility Layer
|
|
*
|
|
* Copyright (C) 2007,2008,2009,2010 Intel Corporation.
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with this library. If not, see <http://www.gnu.org/licenses/>.
|
|
*
|
|
*
|
|
*/
|
|
|
|
#ifndef __COGL_CLIP_STACK_H
|
|
#define __COGL_CLIP_STACK_H
|
|
|
|
CoglHandle
|
|
_cogl_clip_stack_new (void);
|
|
|
|
void
|
|
_cogl_clip_stack_push_window_rectangle (CoglHandle handle,
|
|
int x_offset,
|
|
int y_offset,
|
|
int width,
|
|
int height);
|
|
|
|
void
|
|
_cogl_clip_stack_push_rectangle (CoglHandle handle,
|
|
float x_1,
|
|
float y_1,
|
|
float x_2,
|
|
float y_2,
|
|
const CoglMatrix *modelview_matrix);
|
|
|
|
void
|
|
_cogl_clip_stack_push_from_path (CoglHandle handle,
|
|
CoglHandle path,
|
|
const CoglMatrix *modelview_matrix);
|
|
void
|
|
_cogl_clip_stack_pop (CoglHandle handle);
|
|
|
|
void
|
|
_cogl_clip_stack_flush (CoglHandle handle,
|
|
gboolean *stencil_used_p);
|
|
|
|
|
|
/* TODO: we may want to make this function public because it can be
|
|
* used to implement a better API than cogl_clip_stack_save() and
|
|
* cogl_clip_stack_restore().
|
|
*/
|
|
/*
|
|
* _cogl_clip_stack_copy:
|
|
* @handle: A handle to a clip stack
|
|
*
|
|
* Creates a copy of the given clip stack and returns a new handle to
|
|
* it. The data from the original stack is shared with the new stack
|
|
* so making copies is relatively cheap. Modifying the original stack
|
|
* does not affect the new stack.
|
|
*
|
|
* Return value: a new clip stack with the same data as @handle
|
|
*/
|
|
CoglHandle
|
|
_cogl_clip_stack_copy (CoglHandle handle);
|
|
|
|
#endif /* __COGL_CLIP_STACK_H */
|