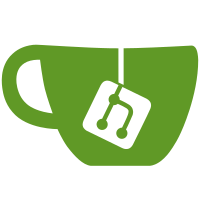
The CoglOutput object represents one output such as a monitor or laptop panel, with information about attributes of the output such as the position of the output within the global coordinate space, and the refresh rate. We don't yet publically export the ability to get output information but we track it for the GLX backend, where we'll use it to track the refresh rate. Reviewed-by: Robert Bragg <robert@linux.intel.com> (cherry picked from commit d7ef9d8d71488d0e6874f1ffc6e48700d5c82a31)
111 lines
2.2 KiB
C
111 lines
2.2 KiB
C
/*
|
|
* Cogl
|
|
*
|
|
* An object oriented GL/GLES Abstraction/Utility Layer
|
|
*
|
|
* Copyright (C) 2012 Red Hat, Inc.
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with this library. If not, see <http://www.gnu.org/licenses/>.
|
|
*
|
|
*
|
|
*/
|
|
|
|
#ifdef HAVE_CONFIG_H
|
|
#include "config.h"
|
|
#endif
|
|
|
|
#include "cogl-output-private.h"
|
|
|
|
#include <string.h>
|
|
|
|
static void _cogl_output_free (CoglOutput *output);
|
|
|
|
COGL_OBJECT_DEFINE (Output, output);
|
|
|
|
CoglOutput *
|
|
_cogl_output_new (const char *name)
|
|
{
|
|
CoglOutput *output;
|
|
|
|
output = g_slice_new0 (CoglOutput);
|
|
output->name = g_strdup (name);
|
|
|
|
return _cogl_output_object_new (output);
|
|
}
|
|
|
|
static void
|
|
_cogl_output_free (CoglOutput *output)
|
|
{
|
|
g_free (output->name);
|
|
|
|
g_slice_free (CoglOutput, output);
|
|
}
|
|
|
|
gboolean
|
|
_cogl_output_values_equal (CoglOutput *output,
|
|
CoglOutput *other)
|
|
{
|
|
return memcmp ((const char *)output + G_STRUCT_OFFSET (CoglOutput, x),
|
|
(const char *)other + G_STRUCT_OFFSET (CoglOutput, x),
|
|
sizeof (CoglOutput) - G_STRUCT_OFFSET (CoglOutput, x)) == 0;
|
|
}
|
|
|
|
int
|
|
cogl_output_get_x (CoglOutput *output)
|
|
{
|
|
return output->x;
|
|
}
|
|
|
|
int
|
|
cogl_output_get_y (CoglOutput *output)
|
|
{
|
|
return output->y;
|
|
}
|
|
|
|
int
|
|
cogl_output_get_width (CoglOutput *output)
|
|
{
|
|
return output->width;
|
|
}
|
|
|
|
int
|
|
cogl_output_get_height (CoglOutput *output)
|
|
{
|
|
return output->height;
|
|
}
|
|
|
|
int
|
|
cogl_output_get_mm_width (CoglOutput *output)
|
|
{
|
|
return output->mm_width;
|
|
}
|
|
|
|
int
|
|
cogl_output_get_mm_height (CoglOutput *output)
|
|
{
|
|
return output->mm_height;
|
|
}
|
|
|
|
CoglSubpixelOrder
|
|
cogl_output_get_subpixel_order (CoglOutput *output)
|
|
{
|
|
return output->subpixel_order;
|
|
}
|
|
|
|
float
|
|
cogl_output_get_refresh_rate (CoglOutput *output)
|
|
{
|
|
return output->refresh_rate;
|
|
}
|