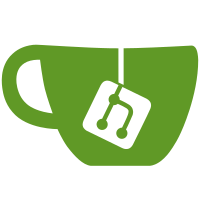
* clutter/clutter-alpha.h: * clutter/clutter-alpha.c: Add a data parameter to the ClutterAlphaFunc; add a data+destroy parameter to clutter_alpha_set_func() and to clutter_alpha_new(), and turned the latter into clutter_alpha_new_full(); add a simple, empty constructor clutter_alpha_new(). These changes makes writing bindings a tad more easy, as bindings require passing their own functions in order to call the real alpha function. * clutter/clutter-behaviour.h: Clean up the header. * clutter/clutter-behaviours.[ch]: * clutter/clutter-behaviour-opacity.[ch]: * clutter/clutter-behaviour-path.[ch]: * clutter/clutter-behaviour-scale.[ch]: Split the ClutterBehaviourPath, ClutterBehaviourOpacity and ClutterBehaviourScale into their own files as they have been growing a bit. Fix ClutterBehaviourPath API. * clutter/clutter-media.h: Remove the commented "metadata_available" signal: gtk-doc chokes up on that. * clutter/clutter-timeline.h: * clutter/clutter-timeline.c: Remove the useless ClutterTimelineAlphaFunc signature; add missing accessor methods for the properties; clean up a bit. * clutter/clutter-util.h: * clutter/clutter-util.c: Remove unneeded function clutter_util_can_create_texture(). * clutter/clutter-feature.h: Sync the name of clutter_feature_get_all() with the name declared in clutter-feature.h. * clutter/Makefile.am: * clutter/clutter.h: Update. * examples/behave.c: Update to the new ClutterAlpha constructor. * examples/super-oh.c: Use the right pointer and avoid the compiler making a fuss about it.
99 lines
3.2 KiB
C
99 lines
3.2 KiB
C
/*
|
|
* Clutter.
|
|
*
|
|
* An OpenGL based 'interactive canvas' library.
|
|
*
|
|
* Authored By Matthew Allum <mallum@openedhand.com>
|
|
* Jorn Baayen <jorn@openedhand.com>
|
|
* Emmanuele Bassi <ebassi@openedhand.com>
|
|
*
|
|
* Copyright (C) 2006 OpenedHand
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with this library; if not, write to the
|
|
* Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
* Boston, MA 02111-1307, USA.
|
|
*/
|
|
|
|
#ifndef _HAVE_CLUTTER_BEHAVIOUR_H
|
|
#define _HAVE_CLUTTER_BEHAVIOUR_H
|
|
|
|
#include <glib-object.h>
|
|
#include <clutter/clutter-alpha.h>
|
|
|
|
G_BEGIN_DECLS
|
|
|
|
#define CLUTTER_TYPE_BEHAVIOUR clutter_behaviour_get_type()
|
|
|
|
#define CLUTTER_BEHAVIOUR(obj) \
|
|
(G_TYPE_CHECK_INSTANCE_CAST ((obj), \
|
|
CLUTTER_TYPE_BEHAVIOUR, ClutterBehaviour))
|
|
|
|
#define CLUTTER_BEHAVIOUR_CLASS(klass) \
|
|
(G_TYPE_CHECK_CLASS_CAST ((klass), \
|
|
CLUTTER_TYPE_BEHAVIOUR, ClutterBehaviourClass))
|
|
|
|
#define CLUTTER_IS_BEHAVIOUR(obj) \
|
|
(G_TYPE_CHECK_INSTANCE_TYPE ((obj), \
|
|
CLUTTER_TYPE_BEHAVIOUR))
|
|
|
|
#define CLUTTER_IS_BEHAVIOUR_CLASS(klass) \
|
|
(G_TYPE_CHECK_CLASS_TYPE ((klass), \
|
|
CLUTTER_TYPE_BEHAVIOUR))
|
|
|
|
#define CLUTTER_BEHAVIOUR_GET_CLASS(obj) \
|
|
(G_TYPE_INSTANCE_GET_CLASS ((obj), \
|
|
CLUTTER_TYPE_BEHAVIOUR, ClutterBehaviourClass))
|
|
|
|
typedef struct _ClutterBehaviour ClutterBehaviour;
|
|
typedef struct _ClutterBehaviourPrivate ClutterBehaviourPrivate;
|
|
typedef struct _ClutterBehaviourClass ClutterBehaviourClass;
|
|
|
|
struct _ClutterBehaviour
|
|
{
|
|
/*< private >*/
|
|
GObject parent;
|
|
ClutterBehaviourPrivate *priv;
|
|
};
|
|
|
|
struct _ClutterBehaviourClass
|
|
{
|
|
GObjectClass parent_class;
|
|
|
|
void (*alpha_notify) (ClutterBehaviour *behave);
|
|
|
|
void (*_clutter_behaviour1) (void);
|
|
void (*_clutter_behaviour2) (void);
|
|
void (*_clutter_behaviour3) (void);
|
|
void (*_clutter_behaviour4) (void);
|
|
void (*_clutter_behaviour5) (void);
|
|
void (*_clutter_behaviour6) (void);
|
|
};
|
|
|
|
GType clutter_behaviour_get_type (void) G_GNUC_CONST;
|
|
|
|
void clutter_behaviour_apply (ClutterBehaviour *behave,
|
|
ClutterActor *actor);
|
|
void clutter_behaviour_remove (ClutterBehaviour *behave,
|
|
ClutterActor *actor);
|
|
void clutter_behaviour_actors_foreach (ClutterBehaviour *behave,
|
|
GFunc func,
|
|
gpointer data);
|
|
ClutterAlpha *clutter_behaviour_get_alpha (ClutterBehaviour *behave);
|
|
void clutter_behaviour_set_alpha (ClutterBehaviour *behave,
|
|
ClutterAlpha *alpha);
|
|
|
|
G_END_DECLS
|
|
|
|
#endif
|