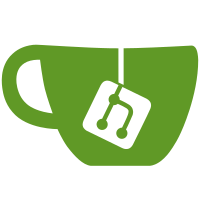
The sub texture backend doesn't work well as a completely general texture backend because for example when rendering with cogl_polygon it needs to be able to tranform arbitrary texture coordinates without reference to the other coordintes. This can't be done when the texture coordinates are a multiple of one because sometimes the coordinate should represent the left or top edge and sometimes it should represent the bottom or top edge. For example if the s coordinates are 0 and 1 then 1 represents the right edge but if they are 1 and 2 then 1 represents the left edge. Instead the sub-textures are now documented not to support coordinates outside the range [0,1]. The coordinates for the sub-region are now represented as integers as this helps avoid rounding issues. The region can no longer be a super-region of the texture as this simplifies the code quite a lot. There are two new texture virtual functions: transform_quad_coords_to_gl - This transforms two pairs of coordinates representing a quad. It will return FALSE if the coordinates can not be transformed. The sub texture backend uses this to detect coordinates that require repeating which causes cogl-primitives to use manual repeating. ensure_non_quad_rendering - This is used in cogl_polygon and cogl_vertex_buffer to inform the texture backend that transform_quad_to_gl is going to be used. The atlas backend migrates the texture out of the atlas when it hits this.
56 lines
1.5 KiB
C
56 lines
1.5 KiB
C
/*
|
|
* Cogl
|
|
*
|
|
* An object oriented GL/GLES Abstraction/Utility Layer
|
|
*
|
|
* Copyright (C) 2009 Intel Corporation.
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with this library; if not, write to the
|
|
* Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
* Boston, MA 02111-1307, USA.
|
|
*/
|
|
|
|
#ifndef __COGL_SUB_TEXTURE_H
|
|
#define __COGL_SUB_TEXTURE_H
|
|
|
|
#include "cogl-handle.h"
|
|
#include "cogl-texture-private.h"
|
|
|
|
#define COGL_SUB_TEXTURE(tex) ((CoglSubTexture *) tex)
|
|
|
|
typedef struct _CoglSubTexture CoglSubTexture;
|
|
|
|
struct _CoglSubTexture
|
|
{
|
|
CoglTexture _parent;
|
|
|
|
CoglHandle full_texture;
|
|
|
|
/* The region represented by this sub-texture */
|
|
gint sub_x;
|
|
gint sub_y;
|
|
gint sub_width;
|
|
gint sub_height;
|
|
};
|
|
|
|
GQuark
|
|
_cogl_handle_sub_texture_get_type (void);
|
|
|
|
CoglHandle
|
|
_cogl_sub_texture_new (CoglHandle full_texture,
|
|
gint sub_x, gint sub_y,
|
|
gint sub_width, gint sub_height);
|
|
|
|
#endif /* __COGL_SUB_TEXTURE_H */
|