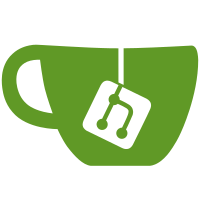
This is the result of running a number of sed and perl scripts over the code to do 90% of the work in converting from 16.16 fixed to single precision floating point. Note: A pristine cogl-fixed.c has been maintained as a standalone utility API so that applications may still take advantage of fixed point if they desire for certain optimisations where lower precision may be acceptable. Note: no API changes were made in Clutter, only in Cogl. Overview of changes: - Within clutter/* all usage of the COGL_FIXED_ macros have been changed to use the CLUTTER_FIXED_ macros. - Within cogl/* all usage of the COGL_FIXED_ macros have been completly stripped and expanded into code that works with single precision floats instead. - Uses of cogl_fixed_* have been replaced with single precision math.h alternatives. - Uses of COGL_ANGLE_* and cogl_angle_* have been replaced so we use a float for angles and math.h replacements.
84 lines
1.7 KiB
C
84 lines
1.7 KiB
C
/*
|
|
* Clutter COGL
|
|
*
|
|
* A basic GL/GLES Abstraction/Utility Layer
|
|
*
|
|
* Authored By Matthew Allum <mallum@openedhand.com>
|
|
*
|
|
* Copyright (C) 2007 OpenedHand
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with this library; if not, write to the
|
|
* Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
* Boston, MA 02111-1307, USA.
|
|
*/
|
|
|
|
#ifndef __COGL_PRIMITIVES_H
|
|
#define __COGL_PRIMITIVES_H
|
|
|
|
typedef struct _floatVec2 floatVec2;
|
|
typedef struct _CoglBezQuad CoglBezQuad;
|
|
typedef struct _CoglBezCubic CoglBezCubic;
|
|
typedef struct _CoglPathNode CoglPathNode;
|
|
|
|
struct _floatVec2
|
|
{
|
|
float x;
|
|
float y;
|
|
};
|
|
|
|
#ifdef CLUTTER_COGL_HAS_GL
|
|
|
|
typedef struct _CoglFloatVec2 CoglFloatVec2;
|
|
|
|
struct _CoglFloatVec2
|
|
{
|
|
GLfloat x;
|
|
GLfloat y;
|
|
};
|
|
|
|
struct _CoglPathNode
|
|
{
|
|
GLfloat x;
|
|
GLfloat y;
|
|
guint path_size;
|
|
};
|
|
|
|
#else /* CLUTTER_COGL_HAS_GL */
|
|
|
|
struct _CoglPathNode
|
|
{
|
|
GLfixed x;
|
|
GLfixed y;
|
|
guint path_size;
|
|
};
|
|
|
|
#endif /* CLUTTER_COGL_HAS_GL */
|
|
|
|
struct _CoglBezQuad
|
|
{
|
|
floatVec2 p1;
|
|
floatVec2 p2;
|
|
floatVec2 p3;
|
|
};
|
|
|
|
struct _CoglBezCubic
|
|
{
|
|
floatVec2 p1;
|
|
floatVec2 p2;
|
|
floatVec2 p3;
|
|
floatVec2 p4;
|
|
};
|
|
|
|
#endif /* __COGL_PRIMITIVES_H */
|