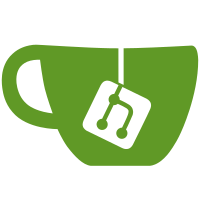
Before flushing the journal there is now a separate iteration that will try to determine if the matrix of the clip stack and the matrix of the rectangle in each entry are on the same plane. If they are it can completely avoid the clip stack and instead manually modify the vertex and texture coordinates to implement the clip. The has the advantage that it won't break up batching if a single clipped rectangle is used in a scene. The software clip is only used if there is no user program and no texture matrices. There is a threshold to the size of the batch where it is assumed that it is worth the cost to break up a batch and program the GPU to do the clipping. Currently this is set to 8 although this figure is plucked out of thin air. To check whether the two matrices are on the same plane it tries to determine if one of the matrices is just a simple translation of the other. In the process of this it also works out what the translation would be. These values can be used to translate the clip rectangle into the coordinate space of the rectangle to be logged. Then we can do the clip directly in the rectangle's coordinate space.
58 lines
1.9 KiB
C
58 lines
1.9 KiB
C
/*
|
|
* Cogl
|
|
*
|
|
* An object oriented GL/GLES Abstraction/Utility Layer
|
|
*
|
|
* Copyright (C) 2007,2008,2009 Intel Corporation.
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with this library. If not, see <http://www.gnu.org/licenses/>.
|
|
*
|
|
*
|
|
*/
|
|
|
|
#ifndef __COGL_JOURNAL_PRIVATE_H
|
|
#define __COGL_JOURNAL_PRIVATE_H
|
|
|
|
#include "cogl-handle.h"
|
|
#include "cogl-clip-stack.h"
|
|
|
|
/* To improve batching of geometry when submitting vertices to OpenGL we
|
|
* log the texture rectangles we want to draw to a journal, so when we
|
|
* later flush the journal we aim to batch data, and gl draw calls. */
|
|
typedef struct _CoglJournalEntry
|
|
{
|
|
CoglPipeline *pipeline;
|
|
int n_layers;
|
|
CoglMatrix model_view;
|
|
CoglClipStack *clip_stack;
|
|
/* Offset into ctx->logged_vertices */
|
|
size_t array_offset;
|
|
/* XXX: These entries are pretty big now considering the padding in
|
|
* CoglPipelineFlushOptions and CoglMatrix, so we might need to optimize this
|
|
* later. */
|
|
} CoglJournalEntry;
|
|
|
|
void
|
|
_cogl_journal_log_quad (const float *position,
|
|
CoglPipeline *pipeline,
|
|
int n_layers,
|
|
CoglHandle layer0_override_texture,
|
|
const float *tex_coords,
|
|
unsigned int tex_coords_len);
|
|
|
|
void
|
|
_cogl_journal_flush (void);
|
|
|
|
#endif /* __COGL_JOURNAL_PRIVATE_H */
|