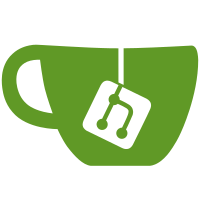
Removes the init() function in favor of executing all environment changes when the file is imported. Additionally ports all unit tests using imports.gi.environment.init() to use the updated module. Part-of: <https://gitlab.gnome.org/GNOME/gnome-shell/-/merge_requests/2822>
148 lines
3.4 KiB
JavaScript
148 lines
3.4 KiB
JavaScript
// -*- mode: js; js-indent-level: 4; indent-tabs-mode: nil -*-
|
|
|
|
// Test cases for SearchResult description match highlighter
|
|
|
|
const JsUnit = imports.jsUnit;
|
|
import Pango from 'gi://Pango';
|
|
|
|
import '../../js/ui/environment.js';
|
|
const Util = imports.misc.util;
|
|
|
|
const tests = [
|
|
{
|
|
input: 'abc cba',
|
|
terms: null,
|
|
output: 'abc cba',
|
|
},
|
|
{
|
|
input: 'abc cba',
|
|
terms: [],
|
|
output: 'abc cba',
|
|
},
|
|
{
|
|
input: 'abc cba',
|
|
terms: [''],
|
|
output: 'abc cba',
|
|
},
|
|
{
|
|
input: 'abc cba',
|
|
terms: ['a'],
|
|
output: '<b>a</b>bc cb<b>a</b>',
|
|
},
|
|
{
|
|
input: 'abc cba',
|
|
terms: ['a', 'a'],
|
|
output: '<b>a</b>bc cb<b>a</b>',
|
|
},
|
|
{
|
|
input: 'CaSe InSenSiTiVe',
|
|
terms: ['cas', 'sens'],
|
|
output: '<b>CaS</b>e In<b>SenS</b>iTiVe',
|
|
},
|
|
{
|
|
input: 'This contains the < character',
|
|
terms: null,
|
|
output: 'This contains the < character',
|
|
},
|
|
{
|
|
input: 'Don\'t',
|
|
terms: ['t'],
|
|
output: 'Don'<b>t</b>',
|
|
},
|
|
{
|
|
input: 'Don\'t',
|
|
terms: ['n\'t'],
|
|
output: 'Do<b>n't</b>',
|
|
},
|
|
{
|
|
input: 'Don\'t',
|
|
terms: ['o', 't'],
|
|
output: 'D<b>o</b>n'<b>t</b>',
|
|
},
|
|
{
|
|
input: 'salt&pepper',
|
|
terms: ['salt'],
|
|
output: '<b>salt</b>&pepper',
|
|
},
|
|
{
|
|
input: 'salt&pepper',
|
|
terms: ['salt', 'alt'],
|
|
output: '<b>salt</b>&pepper',
|
|
},
|
|
{
|
|
input: 'salt&pepper',
|
|
terms: ['pepper'],
|
|
output: 'salt&<b>pepper</b>',
|
|
},
|
|
{
|
|
input: 'salt&pepper',
|
|
terms: ['salt', 'pepper'],
|
|
output: '<b>salt</b>&<b>pepper</b>',
|
|
},
|
|
{
|
|
input: 'salt&pepper',
|
|
terms: ['t', 'p'],
|
|
output: 'sal<b>t</b>&<b>p</b>e<b>p</b><b>p</b>er',
|
|
},
|
|
{
|
|
input: 'salt&pepper',
|
|
terms: ['t', '&', 'p'],
|
|
output: 'sal<b>t</b><b>&</b><b>p</b>e<b>p</b><b>p</b>er',
|
|
},
|
|
{
|
|
input: 'salt&pepper',
|
|
terms: ['e'],
|
|
output: 'salt&p<b>e</b>pp<b>e</b>r',
|
|
},
|
|
{
|
|
input: 'salt&pepper',
|
|
terms: ['&a', '&am', '&', '&'],
|
|
output: 'salt&pepper',
|
|
},
|
|
{
|
|
input: '&&&&&',
|
|
terms: ['a'],
|
|
output: '&&&&&',
|
|
},
|
|
{
|
|
input: '&;&;&;&;&;',
|
|
terms: ['a'],
|
|
output: '&;&;&;&;&;',
|
|
},
|
|
{
|
|
input: '&;&;&;&;&;',
|
|
terms: [';'],
|
|
output: '&<b>;</b>&<b>;</b>&<b>;</b>&<b>;</b>&<b>;</b>',
|
|
},
|
|
{
|
|
input: '&',
|
|
terms: ['a'],
|
|
output: '&<b>a</b>mp;',
|
|
},
|
|
];
|
|
|
|
try {
|
|
for (let i = 0; i < tests.length; i++) {
|
|
let highlighter = new Util.Highlighter(tests[i].terms);
|
|
let output = highlighter.highlight(tests[i].input);
|
|
|
|
JsUnit.assertEquals(`Test ${i + 1} highlight ` +
|
|
`"${tests[i].terms}" in "${tests[i].input}"`,
|
|
output, tests[i].output);
|
|
|
|
let parsed = false;
|
|
try {
|
|
Pango.parse_markup(output, -1, '');
|
|
parsed = true;
|
|
} catch (e) {}
|
|
JsUnit.assertEquals(`Test ${i + 1} is valid markup`, true, parsed);
|
|
}
|
|
} catch (e) {
|
|
if (typeof e.isJsUnitException != 'undefined' &&
|
|
e.isJsUnitException) {
|
|
if (e.comment)
|
|
log(`Error in: ${e.comment}`);
|
|
}
|
|
throw e;
|
|
}
|