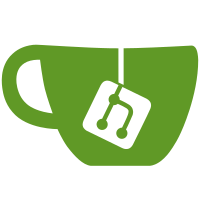
shell_mobile_providers_parse() was returning the country information split into a hash table with providers and a hash table with country names. This patch merges both outputs into a single per-country object, so the parse() method now returns a GHashTable with the following element-type: (element-type utf8 ShellCountryMobileProvider>) This also avoids more complex setups like returning lists inside of hash tables, which was actually breaking either g-i or gtk-doc. shell_mobile_providers_parse() was also modified to allow inputting the paths of the country codes and provider list files to use. If paths are not given, the default ones will be used. This helps us to provide test files during unit tests. Both the findProviderForMCCMNC() and findProviderForSid() methods are exported out of the GSM and CDMA specific classes, and new unit tests for them are implemented. Tests can be run manually with: $> ./tests/run-test.sh tests/unit/mobileProviders.js https://bugzilla.gnome.org/show_bug.cgi?id=687356.
79 lines
2.7 KiB
JavaScript
79 lines
2.7 KiB
JavaScript
// -*- mode: js; js-indent-level: 4; indent-tabs-mode: nil -*-
|
|
|
|
const GLib = imports.gi.GLib;
|
|
const Shell = imports.gi.Shell;
|
|
const JsUnit = imports.jsUnit;
|
|
const ModemManager = imports.misc.modemManager;
|
|
const Environment = imports.ui.environment;
|
|
|
|
Environment.init();
|
|
|
|
// Load test providers table
|
|
let countrycodesPath = GLib.getenv("GNOME_SHELL_TESTSDIR") + "/testcommon/iso3166-test.tab";
|
|
let serviceprovidersPath = GLib.getenv("GNOME_SHELL_TESTSDIR") + "/testcommon/serviceproviders-test.xml";
|
|
let providersTable = Shell.mobile_providers_parse(countrycodesPath, serviceprovidersPath);
|
|
|
|
function assertCountryFound(country_code, expected_country_name) {
|
|
let country = providersTable[country_code];
|
|
JsUnit.assertNotUndefined(country);
|
|
JsUnit.assertEquals(country.get_country_name(), expected_country_name);
|
|
}
|
|
|
|
function assertCountryNotFound(country_code) {
|
|
let country = providersTable[country_code];
|
|
JsUnit.assertUndefined(country);
|
|
}
|
|
|
|
function assertProviderFoundForMCCMNC(mccmnc, expected_provider_name) {
|
|
let provider_name = ModemManager.findProviderForMCCMNC(providersTable, mccmnc);
|
|
JsUnit.assertEquals(provider_name, expected_provider_name);
|
|
}
|
|
|
|
function assertProviderNotFoundForMCCMNC(mccmnc) {
|
|
let provider_name = ModemManager.findProviderForMCCMNC(providersTable, mccmnc);
|
|
JsUnit.assertNull(provider_name);
|
|
}
|
|
|
|
function assertProviderFoundForSid(sid, expected_provider_name) {
|
|
let provider_name = ModemManager.findProviderForSid(providersTable, sid);
|
|
JsUnit.assertEquals(provider_name, expected_provider_name);
|
|
}
|
|
|
|
function assertProviderNotFoundForSid(sid) {
|
|
let provider_name = ModemManager.findProviderForSid(providersTable, sid);
|
|
JsUnit.assertNull(provider_name);
|
|
}
|
|
|
|
// TEST:
|
|
// * Both 'US' and 'ES' country info should be loaded
|
|
assertCountryFound("ES", "Spain");
|
|
assertCountryFound("US", "United States");
|
|
|
|
// TEST:
|
|
// * Country info for 'FR' not given
|
|
assertCountryNotFound("FR");
|
|
|
|
// TEST:
|
|
// * Ensure operator names are found for the given MCC/MNC codes
|
|
assertProviderFoundForMCCMNC("21405", "Movistar (Telefónica)");
|
|
assertProviderFoundForMCCMNC("21407", "Movistar (Telefónica)");
|
|
assertProviderFoundForMCCMNC("310038", "AT&T");
|
|
assertProviderFoundForMCCMNC("310090", "AT&T");
|
|
assertProviderFoundForMCCMNC("310150", "AT&T");
|
|
assertProviderFoundForMCCMNC("310995", "Verizon");
|
|
assertProviderFoundForMCCMNC("311480", "Verizon");
|
|
|
|
// TEST:
|
|
// * Ensure NULL is given for unexpected MCC/MNC codes
|
|
assertProviderNotFoundForMCCMNC("12345");
|
|
|
|
// TEST:
|
|
// * Ensure operator names are found for the given SID codes
|
|
assertProviderFoundForSid(2, "Verizon");
|
|
assertProviderFoundForSid(4, "Verizon");
|
|
assertProviderFoundForSid(5, "Verizon");
|
|
|
|
// TEST:
|
|
// * Ensure NULL is given for unexpected SID codes
|
|
assertProviderNotFoundForSid(1);
|