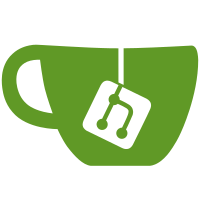
If monitor-changed fires at startup, it will destroy all of the backgrounds, but since this._isStartup is true, won't recreate any of them. Additionally, since _bgManagers is indexed by monitor index, if the primary index is not 0, it could become a sparse array (e.g. [undefined, undefined, primaryBackground]), and our for loop will crash trying to access properties of undefined. Fix both of these issues by always creating background managers for every monitor, hiding them on startup but only showing them after the startup animation is complete. One thing we need to watch out for is that while LayoutManager is constructing, Main.uiGroup / Main.layoutManager will be undefined, so addBackgroundMenu will fail. Fix this by passing down the uiGroup to the background menu code. https://bugzilla.gnome.org/show_bug.cgi?id=709313
69 lines
2.2 KiB
JavaScript
69 lines
2.2 KiB
JavaScript
// -*- mode: js; js-indent-level: 4; indent-tabs-mode: nil -*-
|
|
|
|
const Clutter = imports.gi.Clutter;
|
|
const Lang = imports.lang;
|
|
const St = imports.gi.St;
|
|
const Shell = imports.gi.Shell;
|
|
|
|
const BoxPointer = imports.ui.boxpointer;
|
|
const Main = imports.ui.main;
|
|
const PopupMenu = imports.ui.popupMenu;
|
|
|
|
const BackgroundMenu = new Lang.Class({
|
|
Name: 'BackgroundMenu',
|
|
Extends: PopupMenu.PopupMenu,
|
|
|
|
_init: function(source, layoutManager) {
|
|
this.parent(source, 0, St.Side.TOP);
|
|
|
|
this.addSettingsAction(_("Settings"), 'gnome-control-center.desktop');
|
|
this.addMenuItem(new PopupMenu.PopupSeparatorMenuItem());
|
|
this.addSettingsAction(_("Change Background…"), 'gnome-background-panel.desktop');
|
|
|
|
this.actor.add_style_class_name('background-menu');
|
|
|
|
layoutManager.uiGroup.add_actor(this.actor);
|
|
this.actor.hide();
|
|
}
|
|
});
|
|
|
|
function addBackgroundMenu(actor, layoutManager) {
|
|
let cursor = new St.Bin({ opacity: 0 });
|
|
layoutManager.uiGroup.add_actor(cursor);
|
|
|
|
actor.reactive = true;
|
|
actor._backgroundMenu = new BackgroundMenu(cursor, layoutManager);
|
|
actor._backgroundManager = new PopupMenu.PopupMenuManager({ actor: actor });
|
|
actor._backgroundManager.addMenu(actor._backgroundMenu);
|
|
|
|
function openMenu() {
|
|
let [x, y] = global.get_pointer();
|
|
cursor.set_position(x, y);
|
|
actor._backgroundMenu.open(BoxPointer.PopupAnimation.NONE);
|
|
}
|
|
|
|
let clickAction = new Clutter.ClickAction();
|
|
clickAction.connect('long-press', function(action, actor, state) {
|
|
if (state == Clutter.LongPressState.QUERY)
|
|
return action.get_button() == 1 && !actor._backgroundMenu.isOpen;
|
|
if (state == Clutter.LongPressState.ACTIVATE) {
|
|
openMenu();
|
|
actor._backgroundManager.ignoreRelease();
|
|
}
|
|
return true;
|
|
});
|
|
clickAction.connect('clicked', function(action) {
|
|
if (action.get_button() == 3)
|
|
openMenu();
|
|
});
|
|
actor.add_action(clickAction);
|
|
|
|
actor.connect('destroy', function() {
|
|
actor._backgroundMenu.destroy();
|
|
actor._backgroundMenu = null;
|
|
actor._backgroundManager = null;
|
|
|
|
cursor.destroy();
|
|
});
|
|
}
|