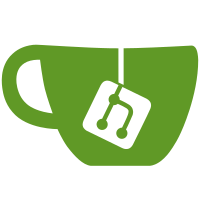
Having StDrawingArea use ClutterCairoTexture causes circularity problems with sizing - StDrawingArea wants to use its allocation for the size of the texture, but ClutterTexture wants to use the size of the texture to determine the requited size. Avoid this by making StDrawingArea directly use Cairo and CoglTexture; while doing this, the API is changed a bit for simplicity and to match our use case: - Instead of clutter_cairo_texture_create(), we have st_drawing_area_get_context() to retrieve an already created context. This can only be called in the ::repaint signal. - The ::redraw signal is changed to ::repaint so we can have st_drawing_area_queue_repaint() that doesn't collide with clutter_actor_queue_redraw() - ::repaint is now emitted lazily when painting the actor rather than synchronously at various different points. https://bugzilla.gnome.org/show_bug.cgi?id=611750
43 lines
1.6 KiB
C
43 lines
1.6 KiB
C
/* -*- mode: C; c-file-style: "gnu"; indent-tabs-mode: nil; -*- */
|
|
#ifndef __ST_DRAWING_AREA_H__
|
|
#define __ST_DRAWING_AREA_H__
|
|
|
|
#include "st-widget.h"
|
|
#include <cairo.h>
|
|
|
|
#define ST_TYPE_DRAWING_AREA (st_drawing_area_get_type ())
|
|
#define ST_DRAWING_AREA(obj) (G_TYPE_CHECK_INSTANCE_CAST ((obj), ST_TYPE_DRAWING_AREA, StDrawingArea))
|
|
#define ST_DRAWING_AREA_CLASS(klass) (G_TYPE_CHECK_CLASS_CAST ((klass), ST_TYPE_DRAWING_AREA, StDrawingAreaClass))
|
|
#define ST_IS_DRAWING_AREA(obj) (G_TYPE_CHECK_INSTANCE_TYPE ((obj), ST_TYPE_DRAWING_AREA))
|
|
#define ST_IS_DRAWING_AREA_CLASS(klass) (G_TYPE_CHECK_CLASS_TYPE ((klass), ST_TYPE_DRAWING_AREA))
|
|
#define ST_DRAWING_AREA_GET_CLASS(obj) (G_TYPE_INSTANCE_GET_CLASS ((obj), ST_TYPE_DRAWING_AREA, StDrawingAreaClass))
|
|
|
|
typedef struct _StDrawingArea StDrawingArea;
|
|
typedef struct _StDrawingAreaClass StDrawingAreaClass;
|
|
|
|
typedef struct _StDrawingAreaPrivate StDrawingAreaPrivate;
|
|
|
|
struct _StDrawingArea
|
|
{
|
|
StWidget parent;
|
|
|
|
StDrawingAreaPrivate *priv;
|
|
};
|
|
|
|
struct _StDrawingAreaClass
|
|
{
|
|
StWidgetClass parent_class;
|
|
|
|
void (*repaint) (StDrawingArea *area);
|
|
};
|
|
|
|
GType st_drawing_area_get_type (void) G_GNUC_CONST;
|
|
|
|
void st_drawing_area_queue_repaint (StDrawingArea *area);
|
|
cairo_t *st_drawing_area_get_context (StDrawingArea *area);
|
|
void st_drawing_area_get_surface_size (StDrawingArea *area,
|
|
guint *width,
|
|
guint *height);
|
|
|
|
#endif /* __ST_DRAWING_AREA_H__ */
|