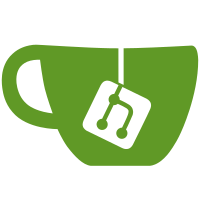
The way the window dimmer shader is applied will cause rendering errors with the rounded corners, invisible borders or shaped textures since it doesn't deal well with the multitexturing used by the MetaShapedTexture. Use an off-screen buffer to flatten the texture before being applied. https://bugzilla.gnome.org/show_bug.cgi?id=659302
29 lines
966 B
GLSL
29 lines
966 B
GLSL
#version 110
|
|
uniform sampler2D tex;
|
|
uniform float fraction;
|
|
uniform float height;
|
|
const float c = -0.2;
|
|
const float border_max_height = 60.0;
|
|
|
|
mat4 contrast = mat4 (1.0 + c, 0.0, 0.0, 0.0,
|
|
0.0, 1.0 + c, 0.0, 0.0,
|
|
0.0, 0.0, 1.0 + c, 0.0,
|
|
0.0, 0.0, 0.0, 1.0);
|
|
vec4 off = vec4(0.633, 0.633, 0.633, 0);
|
|
void main()
|
|
{
|
|
vec4 color = texture2D(tex, cogl_tex_coord_in[0].xy);
|
|
float y = height * cogl_tex_coord_in[0].y;
|
|
|
|
// To reduce contrast, blend with a mid gray
|
|
cogl_color_out = color * contrast - off * c;
|
|
|
|
// We only fully dim at a distance of BORDER_MAX_HEIGHT from the edge and
|
|
// when the fraction is 1.0. For other locations and fractions we linearly
|
|
// interpolate back to the original undimmed color.
|
|
cogl_color_out = color + (cogl_color_out - color) * min(y / border_max_height, 1.0);
|
|
cogl_color_out = color + (cogl_color_out - color) * fraction;
|
|
|
|
cogl_color_out *= color.a;
|
|
}
|