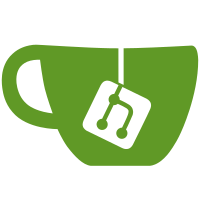
Standard javascript now has Object.assign() which is very similar to Params.parse(), except that the latter by default disallows "extra" parameters. We can still leverage the standard API by simply implementing the error check, and then call out to Object.assign() for the actual parameter merging. https://gitlab.gnome.org/GNOME/gnome-shell/merge_requests/612
26 lines
919 B
JavaScript
26 lines
919 B
JavaScript
// -*- mode: js; js-indent-level: 4; indent-tabs-mode: nil -*-
|
|
|
|
// parse:
|
|
// @params: caller-provided parameter object, or %null
|
|
// @defaults-provided defaults object
|
|
// @allowExtras: whether or not to allow properties not in @default
|
|
//
|
|
// Examines @params and fills in default values from @defaults for
|
|
// any properties in @defaults that don't appear in @params. If
|
|
// @allowExtras is not %true, it will throw an error if @params
|
|
// contains any properties that aren't in @defaults.
|
|
//
|
|
// If @params is %null, this returns the values from @defaults.
|
|
//
|
|
// Return value: a new object, containing the merged parameters from
|
|
// @params and @defaults
|
|
function parse(params = {}, defaults, allowExtras) {
|
|
if (!allowExtras) {
|
|
for (let prop in params)
|
|
if (!(prop in defaults))
|
|
throw new Error(`Unrecognized parameter "${prop}"`);
|
|
}
|
|
|
|
return Object.assign(defaults, params);
|
|
}
|