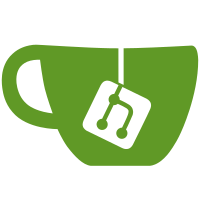
Components are pieces of the shell code that can be added/removed at runtime, like extension, but are tied more directly to a session mode. The session polkit agent, the network agent, autorun/automount, are all components, keyring, recorder and telepathy client are all now copmonents. https://bugzilla.gnome.org/show_bug.cgi?id=683156
59 lines
2.1 KiB
JavaScript
59 lines
2.1 KiB
JavaScript
|
|
const Lang = imports.lang;
|
|
|
|
const Gio = imports.gi.Gio;
|
|
const Meta = imports.gi.Meta;
|
|
const Shell = imports.gi.Shell;
|
|
|
|
const Recorder = new Lang.Class({
|
|
Name: 'Recorder',
|
|
|
|
_init: function() {
|
|
this._recorderSettings = new Gio.Settings({ schema: 'org.gnome.shell.recorder' });
|
|
this._desktopLockdownSettings = new Gio.Settings({ schema: 'org.gnome.desktop.lockdown' });
|
|
this._bindingSettings = new Gio.Settings({ schema: 'org.gnome.shell.keybindings' });
|
|
this._recorder = null;
|
|
},
|
|
|
|
enable: function() {
|
|
global.display.add_keybinding('toggle-recording',
|
|
this._bindingSettings,
|
|
Meta.KeyBindingFlags.NONE, Lang.bind(this, this._toggleRecorder));
|
|
},
|
|
|
|
disable: function() {
|
|
global.display.remove_keybinding('toggle-recording');
|
|
},
|
|
|
|
_ensureRecorder: function() {
|
|
if (this._recorder == null)
|
|
this._recorder = new Shell.Recorder({ stage: global.stage });
|
|
return this._recorder;
|
|
},
|
|
|
|
_toggleRecorder: function() {
|
|
let recorder = this._ensureRecorder();
|
|
if (recorder.is_recording()) {
|
|
recorder.close();
|
|
Meta.enable_unredirect_for_screen(global.screen);
|
|
} else if (!desktopLockdownSettings.get_boolean('disable-save-to-disk')) {
|
|
// read the parameters from GSettings always in case they have changed
|
|
recorder.set_framerate(recorderSettings.get_int('framerate'));
|
|
/* Translators: this is a filename used for screencast recording */
|
|
// xgettext:no-c-format
|
|
recorder.set_filename(_("Screencast from %d %t") + '.' + recorderSettings.get_string('file-extension'));
|
|
let pipeline = recorderSettings.get_string('pipeline');
|
|
|
|
if (!pipeline.match(/^\s*$/))
|
|
recorder.set_pipeline(pipeline);
|
|
else
|
|
recorder.set_pipeline(null);
|
|
|
|
Meta.disable_unredirect_for_screen(global.screen);
|
|
recorder.record();
|
|
}
|
|
}
|
|
});
|
|
|
|
const Component = Recorder;
|